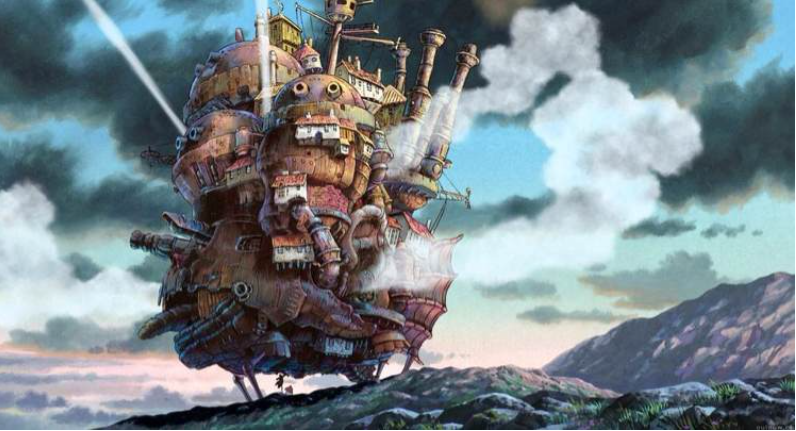
圖片來源: https://inmywordz.com/archives/504
當路由越寫越多變成霍爾的移動城堡一樣雜亂肥大時,自己都看得眼花撩亂啦~考慮使用模組化的概念來統整自己的後端路由是很好的選擇,那麼就來開始吧!
Express.js 4.0 Router 的誕生
Express 輕量框架提供很多整理路由的方式,Express.js 4.0 導入了新的 Router 功能,可以更彈性的撰寫子路由規則以及中介軟體啦!
Express.js 4.0 有加入一個新的 Router 功能,它就像一個迷你的應用程式,可以讓應用程式內部的路由撰寫更方便、更有彈性。
Express.js 在 4.0 版中有許多新的功能,其中一項主要的功能就是 Router,以下我們介紹如何使用 Router 功能來撰寫應用程式。
https://blog.gtwang.org/programming/learn-to-use-the-new-router-in-expressjs-4/
app.use() 產生父路由與路由頭中介
app.use(); mounts middleware for all routes of the app (or those matching the routes specified if you use app.use(‘/ANYROUTESHERE’, yourMiddleware());).
app.use() 是啟用一個路由頭(父路由),並且只要符合該路由頭的每一個子路由,該中介軟體都會啟用,很適合用在登入後驗證是否有登入的會員,是否有訪問路由頁面的權限,以撰寫一些驗證信息。
router.methods() 產生子路由
舊的子路由寫法
1 | const express = require('express'); |
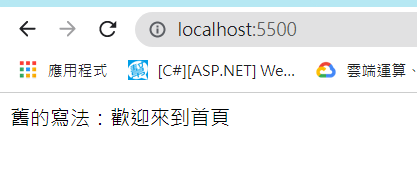
新的子路由寫法
1 | const express = require('express'); |
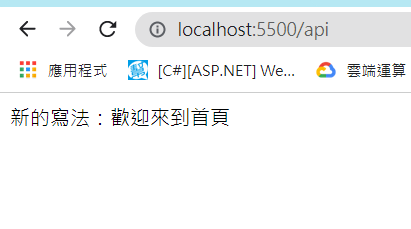
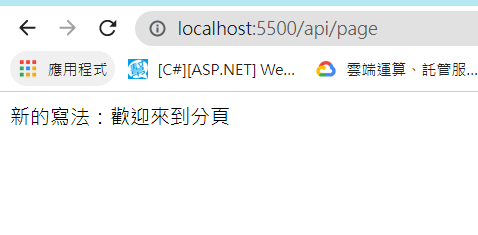
我自己是看不太出來差別,不過文章都是寫道 router 就像一個小型 app 提供你整理路由系統更好的方法,而具體的差異如下:
“A Router doesn’t .listen() for requests on its own”. That might be the main difference.
It’s useful for separating your application into multiple modules – creating a Router in each that the app can require() and .use() as middleware.
- Router 不能單個路由做 port 監聽
- Router 可以使用 require() 與 use() 模組化管理子路由
哪一種比較好眾說紛紜,基本上能適合專案模式的都是好方法。
router.use(); mounts middleware for the routes served by the specific router
router.get is only for defining subpaths.
Consider this example:If you open /first/sud, then the smaller function will get called. If you open /first/user, then the bigger function will get called. In short, app.use('/first', router) mounts the middleware at path /first, then router.get sets the subpath accordingly. https://stackoverflow.com/questions/27227650/difference-between-app-use-and-router-use-in-express
1
2
3
4
5var router = express.Router();
app.use('/first', router); // Mount the router as middleware at path /first
router.get('/sud', smaller);
router.get('/user', bigger);
router.use() 子路由中介
router.use() 是產生子路由中介(middleware)的方式:
在使用 middleware 時必須要注意他的放置位置必須要在 routes 之前,程式在執行的時候會依據 middleware 與 routes 的先後順序來執行,如果不小心將 middleware 放在 routes 之後,那麼在 routes 處理完請求之後就會結束處理的流程,這樣 middleware 就根本不會執行。
https://blog.gtwang.org/programming/learn-to-use-the-new-router-in-expressjs-4/
1 | // 中介軟體要寫在 router 上面 |
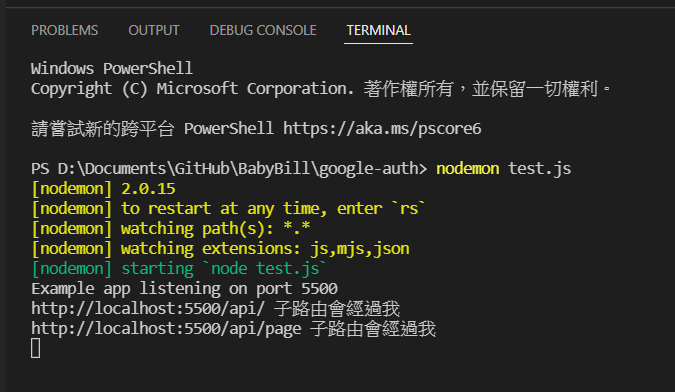
router 模組化
子路由模塊
將幾個子路徑依功能切開可以更方便的統整路由:
1 | const express = require('express'); |
引用模塊
在入口引入模塊:
1 | const express = require('express'); |
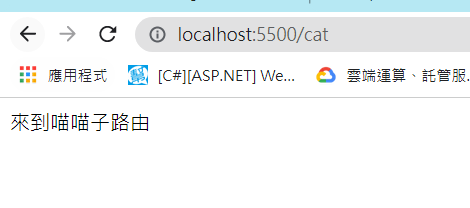
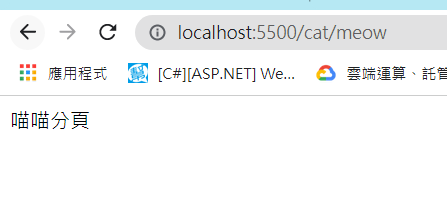
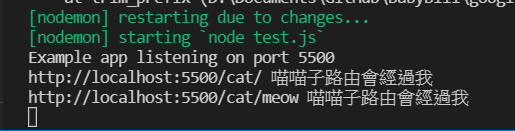
參考文章:
https://stackoverflow.com/questions/27227650/difference-between-app-use-and-router-use-in-express
https://blog.gtwang.org/programming/learn-to-use-the-new-router-in-expressjs-4/
http://expressjs.com/zh-tw/api.html#req
評論